Tech
OpenTofu Foundations: Multi-Environment Management with OpenTofu (Part 7)
Learn how to incorporate testing and validation into your OpenTofu workflows. This session will focus on tools and practices for testing your infrastructure code, including syntax validation, resource policy checks, and simulating infrastructure changes before applying them.
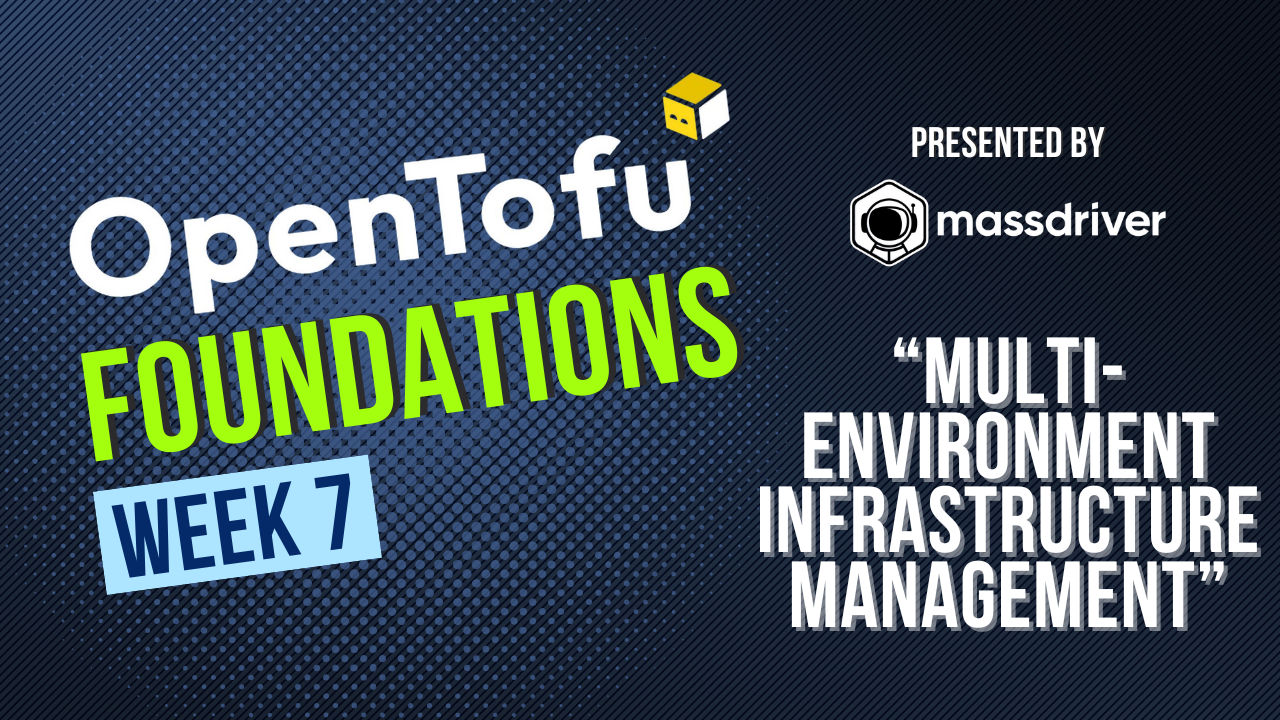